Table of Contents
Motivation
Inspired by automate the boring stuff and motivated by my last automation attempts, I wanted to see if there is more stuff which can be automated.
Creating YouTube thumbnails is one of the time consuming tasks I tried to simplify.
Installation
Pillow is a fork of PIL the Python Imaging Library. We can install it with:
pip install pillow
Loading images
def test_open_image():
thumbnail = Image.open("example_img.jpg")
assert thumbnail.size == (4032, 3024)
Resizing Images
YouTube recommends 1200×720 as the standard thumbnail size. So let’s use pillow to resize the image:
thumbnail = Image.open("example_img.jpg").convert("RGB")
thumbnail.thumbnail((1200, 720), Image.LANCZOS)
thumbnail.save("example_img_resized.jpg")
Loading Fonts
The TrueType font format was developed by Apple and Microsoft as a response to the PostScript font format.
TTF has long been the most common format for fonts on Mac and Windows operating systems.
title_font = ImageFont.truetype(r'c:\windows\fonts\arial.ttf')
If you are sure that the font is a registered system font you don’t need to provide the absolute path
title_font = ImageFont.truetype('arial.ttf')
On macOs you will encounter another related format which is called ttc
title_font = ImageFont.truetype("Supplemental/Futura.ttc")
Font size
The default size of the loaded font is 10. With the size parameter you can adjust that:
title_font = ImageFont.truetype("Supplemental/Futura.ttc", size=120)
Drawing text on images
thumbnail = Image.open("example_img.jpg")
if sys.platform == "win32":
title_font = ImageFont.truetype("arial.ttf", size=120)
else:
title_font = ImageFont.truetype("Supplemental/Futura.ttc", size=120)
draw_surface = ImageDraw.Draw(thumbnail, 'RGBA')
draw_surface.text((0, 0), "Hello Pillow", font=title_font, fill=(148, 14, 76, 0))
thumbnail.save("thumbnail_with_text.jpg")
For adding text we need to add a drawing surface to the image with the Draw function. The text function will draw the text to the surface. The fill parameter takes an RGBA tuple for the font color.
Centering text
To center the text in x-axis we need the width of the image and the width of the textbox
thumbnail = Image.open("example_img.jpg")
thumbnail_width = thumbnail.size[0]
The image object has a size attribute. The width is at index 0
left, top, right, bottom = title_font.getbbox("Hello Pillow")
With the getbbox function we can get the coordinates of the left top and the right bottom of the text’s bounding box so we have to calculate the width with:
textbox_width = right - left
Finally we can calculate the starting position of the textbox:
textbox_start_x = (thumbnail_width - textbox_width) / 2
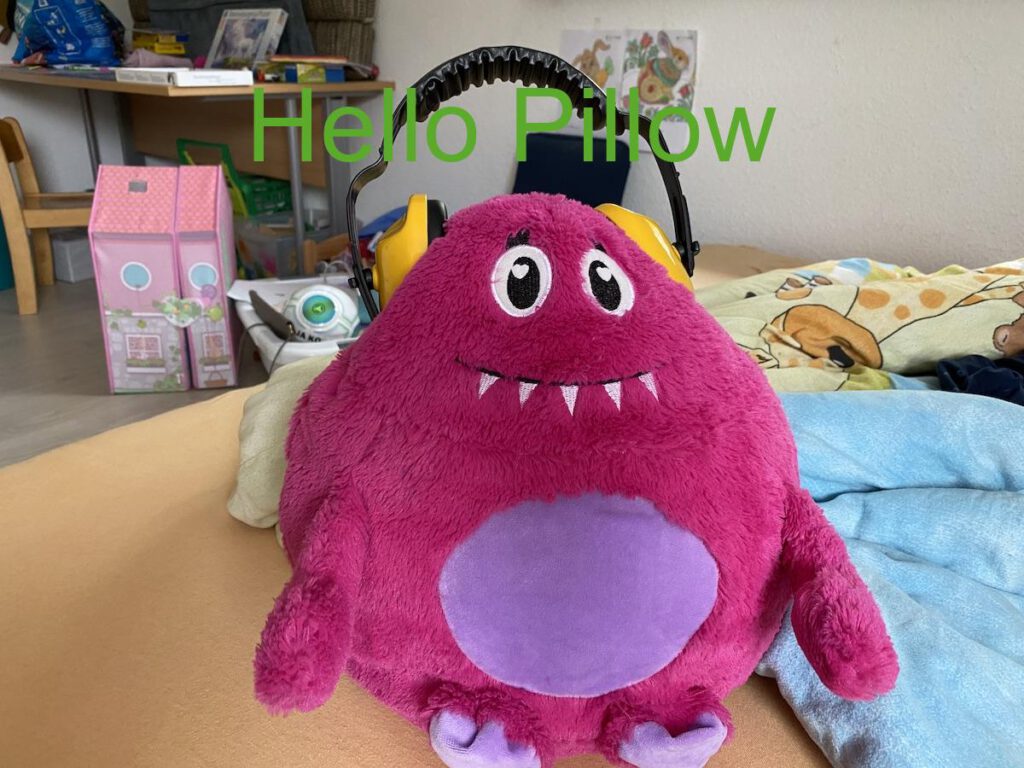
Complete Code
from PIL import Image, ImageFont, ImageDraw
def test_add_centered_text_to_image():
thumbnail = Image.open("example_img.jpg")
draw_surface = ImageDraw.Draw(thumbnail)
title_font = ImageFont.truetype("arial.ttf", size=120)
thumbnail_width = thumbnail.size[0]
left, top, right, bottom = title_font.getbbox("Hello Pillow")
textbox_width = right - left
textbox_start_x = (thumbnail_width - textbox_width) / 2
textbox_start_y = 80
draw_surface.text((textbox_start_x, textbox_start_y), "Hello Pillow", font=title_font, fill=PG_GREEN)
thumbnail.save("thumbnail_with_centered_text.jpg")